We welcome you all to fourth article on Angular Series, before moving forward, let's recap all the concepts we have gone thorough.
A1.) Create A Project — You can check article on creating the project. Click Here.
A2.) Create A Component — You can check the article on creating a component . Click Here.
A3.) Manage-Events — You can check the article on managing basic events such as click. Click Here.
We will look into below concepts 1.) Create angular project -> ng new Angular-Series-A4-Handle Events-Share-Services-Dependency Injection 2.) Create component in angular project -> ng generate component sample-form-component — inline-template — inline-style 3.) Create services in project -> ng g s shared-service 4.) Handle Events 5.) Structural Directives :-> *ngFor
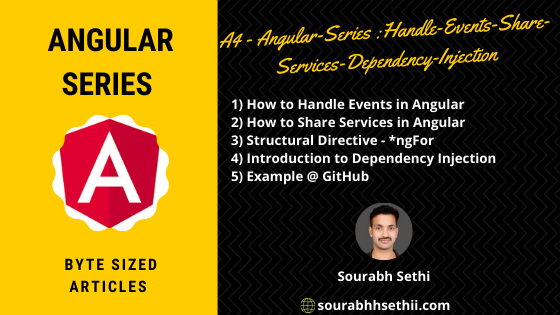
Create Services in Project
Run Below cmd to create service.
ng g s shared-service
g = generate
s = service
shared-service = your custom service name

You can look the service file at below location:
Angular Documentation : https://angular.io/guide/architecture-services
What are services and How it is different from Components?
Services is a classes used to manage specific logic's . It should do something specific and do it well. Angular distinguishes components from services to increase modularity and reusability. By separating a component’s view-related functionality from other kinds of processing, you can make your component classes lean and efficient.
A component can delegate certain tasks to services, such as fetching data from the server, validating user input, or logging directly to the console.
By defining such processing tasks in an injectable service class, you make those tasks available to any component. we can make those services available to components through dependency injection. These services can be shared with multiple components which make inter-component communication possible.
What is dependency Injection?
DI is wired into the Angular framework and used everywhere to provide new components with the services or other things they need. Components consume services; that is, you can inject a service into a component, giving the component access to that service class.
To define a class as a service in Angular, use the @Injectable() decorator to provide the metadata that allows Angular to inject it into a component as a dependency.
@Injectable({
providedIn: ‘root’
})
export class SharedServiceService {
message = ‘You just shared services!!! :)’;
messageObject = [
‘Hello Sourabh, We are doing POC’,
‘Let\’s have another POC on Dependency Injection in Angular’,
‘It will be easy to learn after this article where we discuss DI in details’
];
constructor() { }
}
Use the @Injectable() decorator to indicate that a component or other class (such as another service, a pipe, or an NgModule) has a dependency.
The injector is the main mechanism. Angular creates an application-wide injector for you during the bootstrap process, and additional injectors as needed. You don’t have to create injectors.
An injector creates dependencies, and maintains a container of dependency instances that it reuses if possible.
A provider is an object that tells an injector how to obtain or create a dependency.
For any dependency that you need in your app, you must register a provider with the app’s injector, so that the injector can use the provider to create new instances. For a service, the provider is typically the service class itself.
export class SampleFormComponentComponent implements OnInit {
constructor(private sharedServiceService: SharedServiceService) {
}
}
With the help of DI (Dependency Injector), we can share multiple services with component. We will look DI in detail in next article. These shared services can be accessed in the component as shown in above example. You can inject it in constructor and use the object of that that service to access the method and variables of the class. As shown in example, sharedServiceService object is used to access the messageObject array available available in SharedServiceService Service.
<ul>
<li *ngFor=” let objectinArray of sharedServiceService.messageObject” >
{{objectinArray}}
</li>
</ul>
Manage Event
Events can be managed as shown in below example (#textInput) by using # reference variable.This reference variable’s value can be accessed as textInput.value and can pass into function which will be further invoked by click event such as onClick. You can call any inbuilt event function such, mouseout, mouseleave, mouseenter etc. You can clone the repository to run below demo.
<div> <input #textInput type=”text”> <button (mouseout) = “mouseOutEvent($event)” (click)=”onClick($event,textInput.value);”> Click Event</button> </div>
Directives
Directives create DOM elements and change their structure or behavior in an Angular application. There are three types of directives in Angular:
1.) Components: Directives with templates. 2.) Attribute directives: Change appearance and behavior of anelement, component, or other directive. 3.) Structural directives: Change DOM layout by adding or removing elements.
The basic difference between a component and a directive is thata component has a template, whereas an attribute or structural directive does not have a template. Angular has provided us many inbuilt structural and attribute directives. Inbuilt structural directives are *ngFor and *ngIf. Attribute directives are NgStyle and NgModel.
*ngFor is a structure directive used as for loop on DOM elements .Consider the below example. In this, the *ngFor directive will add messageObject.length time li elements with it’s value.
<ul> <li *ngFor=” let objectinArray of sharedServiceService.messageObject” > {{objectinArray}} </li> </ul>
Angular Documentation : https://angular.io/guide/architecture-components#directives
Repository : You can clone below repository and run the application to check the demo.
Output Screen:
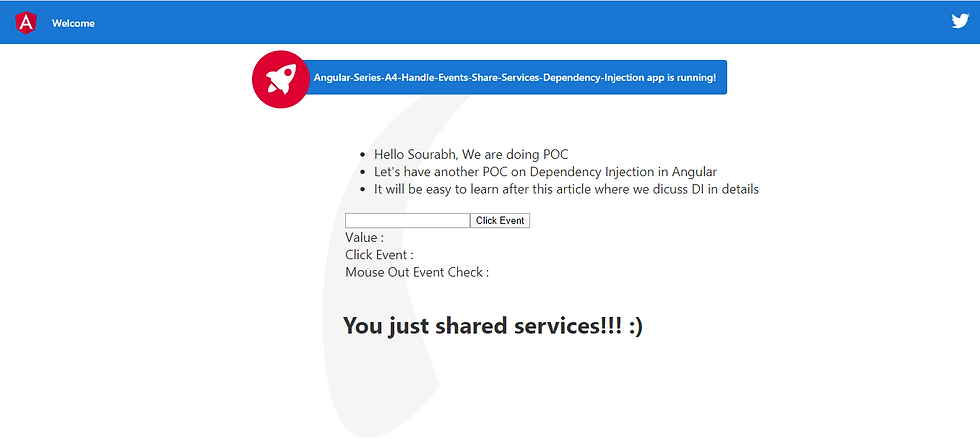
Interview Questions :
Question 1.) How to handle events?
Question 2.) Explain Services and Dependency Injection in brief?
Question 3.) what are the directives?
Question 4.) What are the types of directives?
Question 5.) What are difference between components and services ?
Comments